Stop Writing Crap Code
A practical guide to writing readable and easily debug-able code.
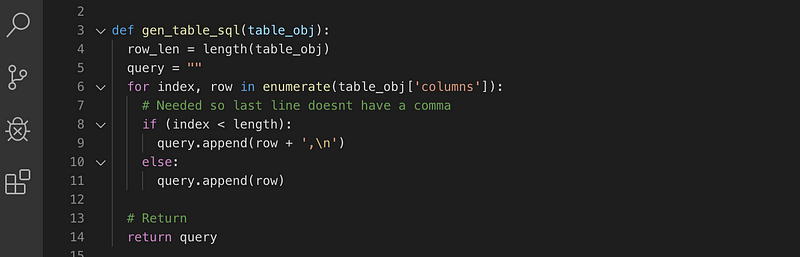
Really, you can stop anytime. Literally right now. You can do it.
Article available in Polish here.
Writing bad code is inevitable. Everyone does it at some point in their life and it’s probably not even their fault. Your typical computer science degree doesn’t teach you what it takes to write clean concise code. Follow a few simple guidelines and writing better code will start to come naturally.
The If Trap
Stop defaulting to using else statements. Taking a little time to think through what you are trying to do can save you time debugging complicated logic later. Take the following for example:if (index < length):
query.append(row + ',\n')
else:
query.append(row)
This isn’t hard to read but add a few more lines of code around it and you start to lose track of what you’re achieving. So here’s a simple clarification where we take out duplicated logic and simplify what we are evaluating:query.append(row)if(index != length):
query.append(',\n')
With a simple refactor, we can now see that we will always append the row. If you do the same thing in both the if
and the else
, move it outside. Additionally, we simplified the comparison. Instead of using the comparison to show all the times the if
is evaluated it now shows the one case where it’s not.
Use Built-In Functionality
This is one of the ones that haunted me for a long time. I wouldn’t take the time to find functions that were built into a language. As a result, my code would have lots in the way of bloat. For example, this is a bit of code that I used at one point to combine all the rows of an array. All the rows needed a comma to separate them except the last one.def gen_table_sql(table_obj):
row_len = length(table_obj)
query = ""
for index, row in enumerate(table_obj['columns']):
query.append(row)
# Needed so last line doesnt have a comma
if (index < length):
query.append(',\n')
return query
I iterated over each row checking along the way to see if I was on the last row of the array. Not terrible code but it takes a moment to read over it and to understand what is being said. Then I looked for five minutes and found I could do the same thing with the string join method. I was able to take that whole bit of logic and turn it into one line:def gen_table_sql(table_obj):
# Joins all rows with a comma and a newline except for the last
query = ',\n'.join(table_obj['columns'])
return query
Stop Hiding Logic in Functions
Following the single responsibility principle we know that we want each function to have one job. In the example above it seems that the function is down to one task. However, it’s hiding secret functionality — the fact that it requires the table_obj to have a property called columns. On top of that, it requires that the columns attribute to be a list.def gen_table_sql(col_list):
# Joins all rows with a comma and a newline except for the last
query = ',\n'.join(col_list)
return query
This is the better way to write this function. You will simply pass in the most simple data possible like this gen_table_sql(table_obj[‘columns’]).
Be Descriptive
The last step in writing better code involves being descriptive. Below I have changed the name of the function to describe what it does. Along with naming well, I added a docstring so that when I am developing I can get a brief description in my editor as seen below:def join_col_list(col_list):
'''Takes in column list and joins with commas to form sql statement'''
query = ',\n'.join(col_list)
return query

That’s It!
These little tips will rocket you on your way to writing great code. Prepare for a huge boost in productivity and regaining your sanity as your code becomes easier to read and write.